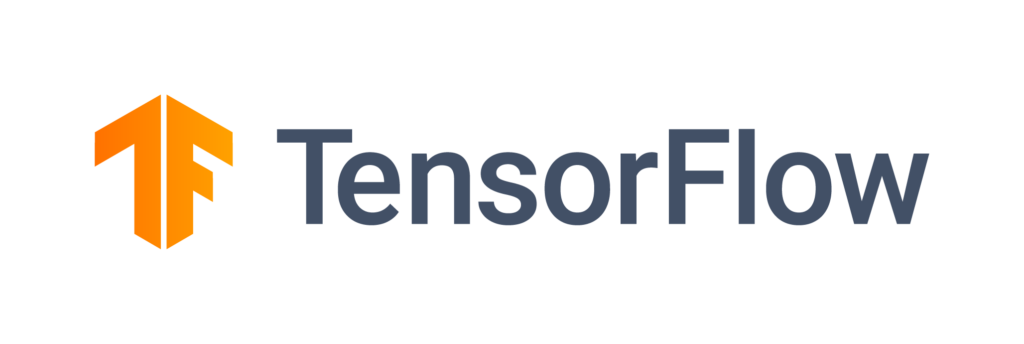
This is the first of several examples that i’ll share with you. In this example you’ll learn how to prepare your environment in order to work wth tensorflow, and also, we will predict a value taking into account a collection of data, using Tensorflow.
WARNING ! : I’ll focus my examples in how to run little projects (practical), but you must search for theorical information (also i’ll add some specific links) in order to help you understand what is behind those calculation examples.
Pre -Requisites
- Linux Machine (I’m using Ubuntu 18.04)
- Python 3 + PIP3 ( + Know what environments are and how to use it)
- VSCode
Create a file named setup-1.sh and paste the code below:
# Python3 sudo apt-get install python3 -y; py3_version=$(python3 --version); echo -e "\e[31m$py3_version\e[39m"; # Pip3 sudo apt-get install python3-pip -y; pip3_version=$(pip3 --version); echo -e "\e[31m$pip3_version\e[39m"; # VSCode sudo snap install vscode --classic;
Be sure that setup-1.sh has 775 permissions (sudo chmod 775 setup-1.sh
).
Execute in your terminal ./setup-1.sh
in order to install required software (tested in Ubuntu 18.04).
Before you put hands on the following example, is better you review the following Videos (better if you follow the order):
Python:
Tensorflow/Machine Learning:
- But what is a Neural Network? | Deep learning, chapter 1
- Gradient descent, how neural networks learn | Deep learning, chapter 2
- Take special attention to Chapter 2 on minutes:
- 03:58 = Mean squared error
- 10:33 = Gradient descent
- Take special attention to Chapter 2 on minutes:
- Optimizers
- Gradient Descent, Step-by-Step (Math analysis-1)
- Stochastic Gradient Descent, Clearly Explained ! (Math analysis-2)
- tf.keras.Sequential
- Shapes and dynamic dimensions
- The meaning of shapes in NumPy
- How to interpret “loss” and “accuracy” for a machine learning model
- Loss vs Accuracy
Python Virtual Environment:
- Create a directory for your new project (called regression-example).
- Inside that directory, create a file called setup-2.sh, and give it execute permissions (
sudo chmod 775 setup-2.sh
). - Also, inside that dir, create a file called requirements.txt
- Execute in your terminal:
./setup-2.sh
Inside requirements.txt you must paste:
tensorflow==2.0.0-alpha0 numpy==1.16
Inside setup-2.sh you must paste:
pip3 install virtualenv; virtualenv PythonEnvironment --python=python3; source PythonEnvironment/bin/activate; pip3 install -r requirements.txt && \ deactivate;
Problem to solve:
Now, after you have reviewed the links, and understood some basic concepts, we can start with our first example.
We need to fit some points (Xs, Ys) to a line. And then use that line (equation) to predict a value (ie. Xs= 10, Ys = ?)
Running the example:
Next, we will create the simplest possible neural network. It has 1 layer, and that layer has 1 neuron (unit=1). The input_shape specifies that the input value will be a simple scalar.
We will use Keras that it’s a high level API for deep learning.
Let’s create a file called hello-world.py and paste the following code:
import tensorflow as tf import numpy as np from tensorflow import keras model = tf.keras.Sequential([keras.layers.Dense(units=1, input_shape=[1])])
model.compile(optimizer='sgd', loss='mean_squared_error') xs = np.array([-1.0, 0.0, 1.0, 2.0, 3.0, 4.0], dtype=float) ys = np.array([-3.0, -1.0, 1.0, 3.0, 5.0, 7.0], dtype=float) model.fit(xs, ys, epochs=5000) print(model.predict([10.0]))
Note: Review http://keras.layers.Dense and tf.keras.optimizers.SGD
To run the code:
Then, in your terminal execute (using the environment):
PythonEnvironment/bin/python3 hello-world.py
Review Results:
After iterations have finished (5000 iterations in this example), the predicted value for Xs=10 is Ys=18.985 (Close to the real value Ys= 19).
You might have thought 19, right? But it ended up being a little under. Why do you think that is?
Remember that neural networks deal with probabilities, so given the data that we fed the NN with, it calculated that there is a very high probability that the relationship between X and Y is Y=2X-1, but with only 6 data points we can’t know for sure. As a result, the result for 10 is very close to 19, but not necessarily 19.
Based in course Introduction to TensorFlow for Artificial Intelligence, Machine Learning, and Deep Learning